Guide to sending Price2Spy API requests with Python
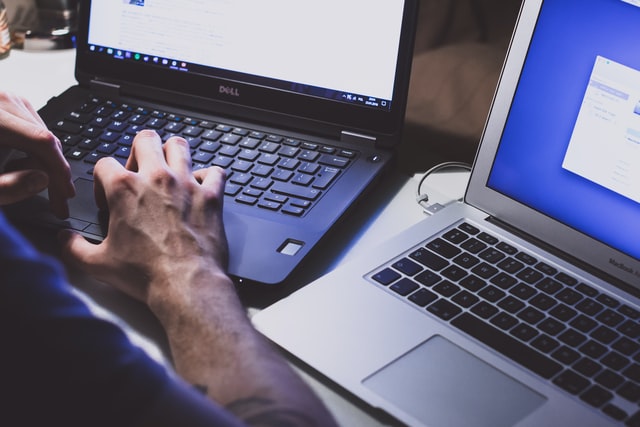
One of the features that Price2Spy prides itself on is definitely API. We are constantly working on improving all features, so that was the case with API as well. Since we know how popular Python is, we decided to allow Price2Spy API requests through Python.
Several clients have also suggested this, and as we are always listening to you, we’ve decided to do something about it.
Therefore, we present you with the guide to sending requests in Price2Spy API through Python.
Before we move on to concrete steps, it is important to note that there are a few requirements:
1. Having Python installed on your computer
We believe you already have it, but just in case you don’t, you can install the latest version of Python from the official website.
2. Having a code editor/environment for sending requests
There are many environments available for Python development to choose from. Due to simplicity, we used Visual Studio Code but you can use any editor you feel comfortable with.
Instructions:
a) Start by importing necessary libraries:
import requests
importbase64
b) Insert the appropriate URL and API key
API_URL = "https://api.price2spy.com/rest/v1/"
API_KEY = "(your API key goes here)"
c) Define a function for decoding the API key:
Another important step is to define a function for decoding the API key. This will, later on, be used to decode the API key in order to properly sent the request.
def decodeAPIKey(key):
api_key_bytes = key.encode()
encoded = base64.b64encode(api_key_bytes)
return(encoded.decode('utf-8'))
d) Setting up the headers
After you’ve performed the previous steps, it’s time for setting up the headers.
headers = {
'accept': 'application/json',
'Content-Type': 'application/json',
'Authorization': 'Basic ' + decodeAPIKey(API_KEY),
}
e) Defining a function for getting the pricing data for a specific category id
The following step is to define a function for getting the pricing data for a specific category id.
def getCurrentPricingData(categoryId):
data = '{ "active": true, "categoryId": ' + str(categoryId) + '}'
response = requests.post(API_URL + 'get-current-pricing-data', headers=headers, data=data)
currentPricingDataResponse = response.json()
return currentPricingDataResponse
f) Sending the actual request and storing the response
This part is responsible for sending the request and storing the response in the variable “response” as serialized JSON
response = requests.get(API_URL + 'get-categories', headers=headers)
g) Deserializing the JSON response
It is necessary to deserialize the JSON response so it becomes a dict:
try:
currentPricingDataResponse = response.json()
print(response.content)
h) Final and the most important step
The final part is the main one. It loops through each category, prints its name, calls the function for current pricing data for that category ID, loops through each product, and prints its name, all website URLs, and prices on them. Also, it will print the error if it arises.
try:
for x in currentPricingDataResponse['category']:
print("Category name: " + x.get('name') + "\n")
currentPricingDataResponse = getCurrentPricingData(x.get('id'))
for y in currentPricingDataResponse["products"]["product"]:
print("Product name: " + y['productName'] + '\n')
#print("Website URL: " + y['urls']['url'][0].get('url'))
#print("Last measured price: " +
str(y['urls']['url'][0]['lastMeasurement']['price']['amount']) + ' ' +
y['urls']['url'][0]['lastMeasurement']['price']['currency'])
for z in y['urls']['url']:
print("Website URL: " + z.get('url'))
print("Last measured price for that website: " +
str(z['lastMeasurement']['price'].get('amount')) + ' '
+z['lastMeasurement']['price'].get('currency'))
print('\n')
print('\n')
except NameError as err:
print("Name error: {0}".format(err))
except:
print("Error occured.")
We hope you find these initial insights useful. In case you have any dilemma regarding this, or you would simply like to use some other way of API integration, we invite you to visit our Price2Spy API Documentation page. There you can find all the instructions that you might need, and we make sure to update it regularly.
As always, if you need any additional help, please don’t hesitate to reach out – our team would be happy to help you!